Integrating SEO considerations into web development projects
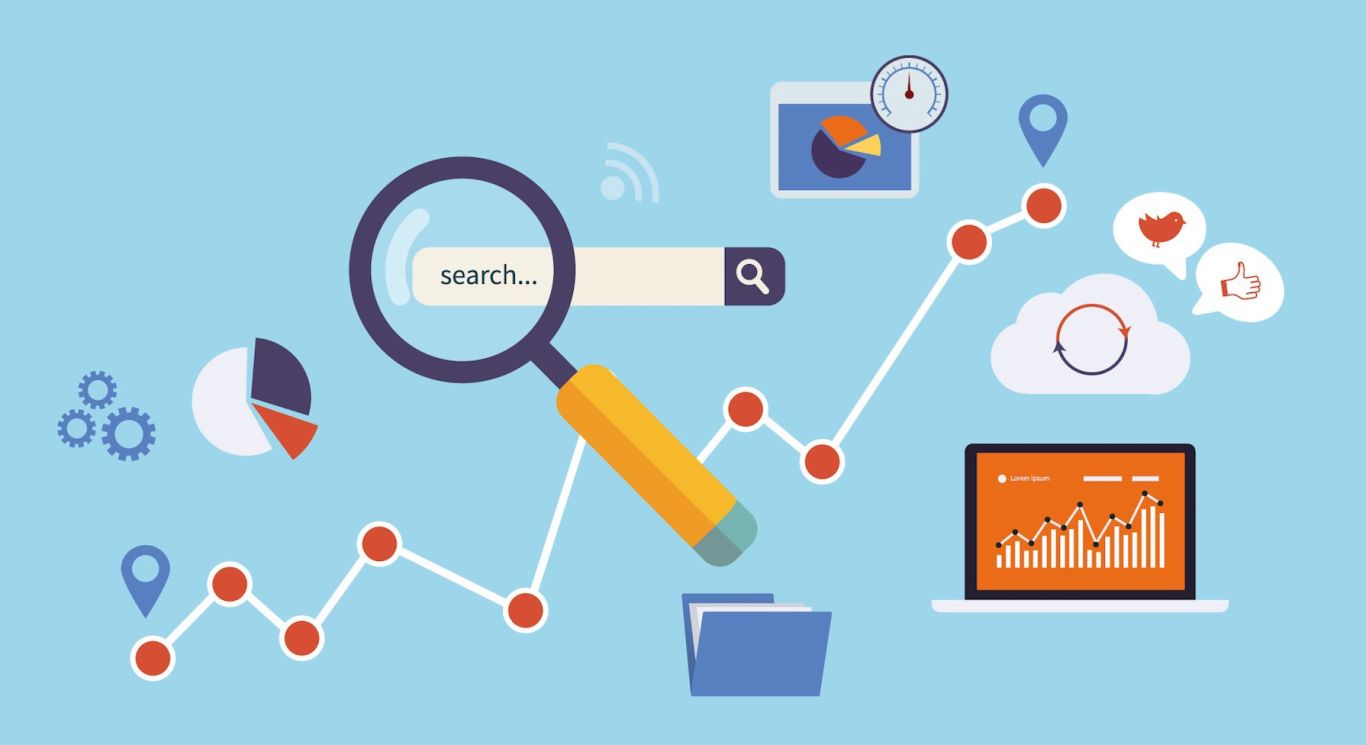
🚀 The easy way to Integrating SEO into ASP.NET Core Projects (with Code You Don't Have to Write)
Search engine optimization isn’t just a job for marketers. If you're building modern web apps, SEO is part of your development workflow—and it should be treated as such. When you're on ASP.NET Core, there are smart ways to bake SEO into your site’s foundation using clean architecture, strong conventions, and… a bit of help from someone who’s already done it.
This guide shares key technical SEO strategies for ASP.NET Core developers, plus real-world examples pulled from my consulting toolkit — a ready-to-use, battle-tested codebase for rapidly building high-performing, SEO-friendly web apps.
🔍 1. Semantic HTML in Razor Views
Semantic HTML improves accessibility and crawlability. In Razor Pages or MVC Views:
<main>
<article>
<h1>@Model.Title</h1>
<p>@Model.Description</p>
</article>
</main>
Avoid using <div>
or <span>
as structural tags unless necessary. This approach mirrors how content is understood by screen readers and search engines.
✅ Already scaffolded in my DevStack layout templates.
🧭 2. SEO-Friendly URLs with Slugs
Use descriptive, keyword-rich slugs instead of cryptic IDs.
[Route("articles/{slug}")]
public IActionResult Article(string slug) => View();
Auto-generate slugs like so:
public static string Slugify(string input)
{
return Regex.Replace(input.ToLowerInvariant(), @"[^a-z0-9]+", "-").Trim('-');
}
✅ Included as part of my URL routing helper utilities.
⚙️ 3. Meta Tags, Titles & Open Graph
In your _Layout.cshtml
:
<title>@ViewData["Title"] - My Site</title>
<meta name="description" content="@ViewData["Description"]" />
<meta property="og:image" content="@ViewData["OgImage"]" />
From controller or Razor Page:
ViewData["Title"] = article.Title;
ViewData["Description"] = article.Summary;
✅ Already built into the view model pipeline of DevStack — just set the values and go.
📚 4. Structured Data with JSON-LD
Schema markup enhances how your content appears in search results:
<script type="application/ld+json">
{
"@context": "https://schema.org",
"@type": "Article",
"headline": "@Model.Title",
"author": { "@type": "Person", "name": "@Model.Author" },
"datePublished": "@Model.PublishedDate.ToString("yyyy-MM-dd")"
}
</script>
✅ Schema types handled dynamically with prebuilt partials in DevStack.
⚡ 5. Performance and Core Web Vitals
ASP.NET Core gives you tools to keep your site fast:
app.UseResponseCompression();
Other essentials:
- ✅ Responsive image handling
- ✅ Thumbnail generation
- ✅ CDN-aware cache headers
- ✅ Bundling/minification via Gulp or Webpack
✅ DevStack comes wired for Lighthouse compliance.
📸 6. Image SEO + Sitemaps
Image SEO is often overlooked — don’t be that developer.
In your image handling pipeline:
jpeg.Title = download.Name;
jpeg.Subject = download.Description?.Truncate(100);
jpeg.Creator = download.Author;
Generate and serve an image sitemap:
return Content(SitemapBuilder.GenerateImageSitemap(), "application/xml");
✅ Fully implemented in DevStack — no external tools required.
📱 7. Mobile-First Responsive Design
<meta name="viewport" content="width=device-width, initial-scale=1">
- Uses Bootstrap/Tailwind for layout
- Fluid typography and image scaling
✅ The mobile UX is baked in. It just works.
🧾 8. Canonical URLs & Redirects
Prevent duplicate content:
<link rel="canonical" href="@Context.Request.GetDisplayUrl()" />
Redirect middleware handles:
- HTTPS enforcement
- Lowercasing URLs
- Trailing slash normalization
✅ Middleware included out of the box.
🤖 9. Robots, Crawlers & Indexing
- Serve up
robots.txt
fromwwwroot
- Submit
sitemap.xml
via Google Search Console - Avoid
noindex
ornofollow
errors
✅ Tools included to automate and validate robot behavior.
🛡️ 10. Logging SEO Issues
Use Serilog for:
- 404 tracking
- Missing metadata
- Bot access logs
✅ My GlobalErrorHandlerService + Serilog SQL sink = zero surprises.
✨ Bonus: Rich Text Editor with Markdown & Schema
Need authors or editors to add blog content? DevStack includes a rich text editor with custom Markdown-like syntax, support for:
- Inline code
- Tables
- Annotations
- Images
- Popups
All of which safely convert to semantic HTML with schema support.
✅ This means you can write SEO-optimized blogs without touching raw HTML.
🎯 Want This Done for You?
If you’ve read this far, you probably care about technical SEO and .NET development. I do too.
Instead of building all this from scratch, you can leverage my DevStack—a proven ASP.NET Core 8 codebase with everything in this post prebuilt.
Need something custom? Let’s talk. I offer:
- Consulting
- Licensing
- Implementation help
- Custom integrations
💡 Because you could spend weeks building all this… or plug it in today.