Better “Did You Mean?” in .NET Core 8
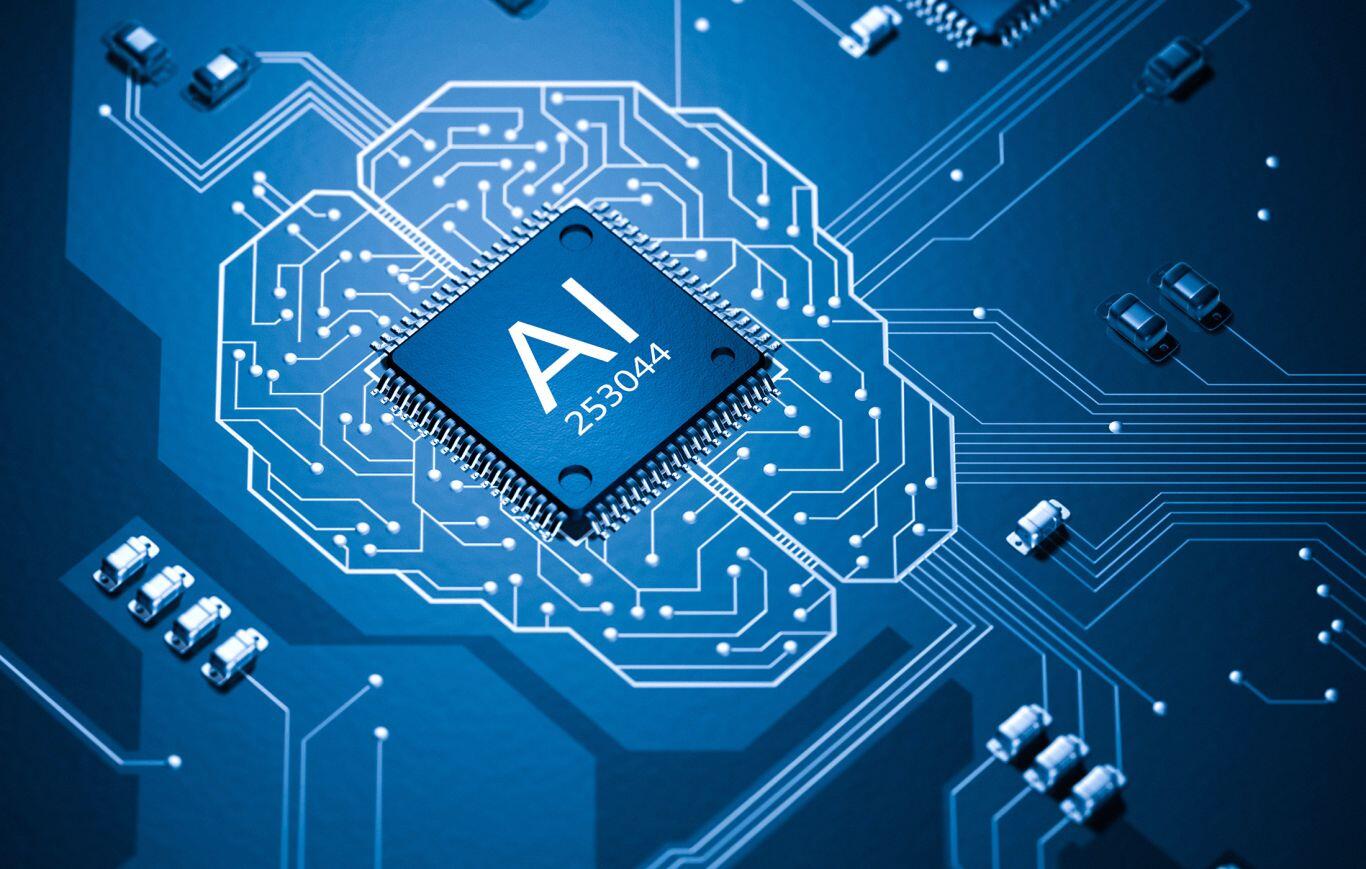
To build a modern, typo-tolerant search system in ASP.NET Core 8, you’d want to go beyond SOUNDEX()
with one or more of these:
✅ 1. Levenshtein Distance (Edit Distance)
Levenshtein measures how many single-character edits (insertions, deletions, substitutions) are needed to change one word into another.
How to use in .NET Core:
Use the FuzzySharp
NuGet package (a .NET port of Python's fuzzywuzzy
):
dotnet add package FuzzySharp
using FuzzySharp;
int score = Fuzz.Ratio("Jonson", "Johnson"); // Score between 0–100
if (score > 85) {
// Suggest this match
}
It supports partial matches, token sets, etc. Great for building suggestions.
✅ 2. Trigram Search with EF Core / SQL Server
Use trigrams (3-letter substrings) to build a similarity index.
If you're using PostgreSQL, trigram search is built-in with pg_trgm
.
In SQL Server, you’d roll your own via:
- Preprocessing text into trigrams
- Storing them in a related table
- Querying with overlap counts
You can also calculate similarity by common trigram count.
✅ 3. Full-Text Search
Use SQL Server’s built-in Full-Text Indexing to allow:
- Stemming
- Inflectional forms
- Synonyms
- Proximity queries
For fuzzy or partial matches, combine this with Levenshtein in the app layer.
✅ 4. ElasticSearch or Azure Cognitive Search
These engines offer:
- Out-of-the-box fuzzy search
- Synonym maps
- Auto-complete with typo tolerance
- Ranking and scoring
If your app needs robust multilingual or NLP-powered search, Elastic or Azure Search is ideal.
✅ 5. Custom Dictionary + Suggestions
Keep a table of common search terms or names and use similarity scoring (Levenshtein or FuzzySharp
) to suggest:
var suggestions = dictionary
.Where(term => Fuzz.Ratio(term, userInput) > 80)
.OrderByDescending(term => Fuzz.Ratio(term, userInput));
This is the simplest, fastest way to create a “Did you mean?” engine.
🧠 What I’ve Already Built
In my DevStack, I’ve done this before:
- Fuzzy lookup for people and artists
- Smart search over downloadable files
- Integrated fallback suggestions (
Did you mean...?
) for misspelled terms - Seamless indexing and caching for fast results
And of course, it all plays nicely with routing, JSON-LD, and SEO features. You can skip the plumbing and jump to results.
🚀 Ready to Upgrade Search?
If your users are typing in names, titles, products, or any variable content—let’s build a search that understands them.
→ Book a quick discovery call and I’ll show you how to turn Core 8 + fuzzy logic into real business results.
You can also explore my tech stack or business experience if you want to know how I’ve built these systems for real-world clients.
Sample Code
Here are practical code samples for each fuzzy logic strategy in .NET Core 8, tailored for your use case and stack:
✅ 1. EF Core + Levenshtein Distance (in-memory search)
Best for short lists (names, tags, filenames) where performance isn't a bottleneck.
Install FuzzySharp
:
dotnet add package FuzzySharp
C# Sample: In-memory fuzzy match from DB
using FuzzySharp;
using Microsoft.EntityFrameworkCore;
// Assume `term` is your user input
public async Task<List<string>> GetFuzzyMatchesAsync(string term)
{
var candidates = await _context.Downloads
.Select(d => d.Title)
.ToListAsync();
return candidates
.Where(c => Fuzz.Ratio(c, term) > 80)
.OrderByDescending(c => Fuzz.Ratio(c, term))
.Take(5)
.ToList();
}
🔍 2. ElasticSearch Fuzzy Search Integration
Best for smart, typo-tolerant, scalable search (documents, blogs, files, etc.)
You’ll need:
- Elasticsearch.NET
- Or high-level NEST client
Install:
dotnet add package NEST
Indexing Example:
var settings = new ConnectionSettings(new Uri("http://localhost:9200")).DefaultIndex("downloads");
var client = new ElasticClient(settings);
await client.IndexDocumentAsync(new {
id = 1,
title = "Guitar Chord Book",
description = "A PDF of all major and minor chords"
});
Fuzzy Search Query:
var response = await client.SearchAsync<dynamic>(s => s
.Query(q => q
.Fuzzy(fz => fz
.Field("title")
.Value("gittar")
.Fuzziness(Fuzziness.Auto)
)
)
);
Response hits are typo-tolerant and sorted by score.
✨ 3. Custom Trigram Matching in SQL Server
Useful for short text fields like titles, names.
Step 1: Create a table to store trigrams
CREATE TABLE Trigrams (
Id INT,
Trigram NVARCHAR(10)
);
Step 2: Function to extract trigrams
CREATE FUNCTION dbo.GetTrigrams(@text NVARCHAR(MAX))
RETURNS @result TABLE (Trigram NVARCHAR(10))
AS
BEGIN
DECLARE @i INT = 1;
WHILE @i <= LEN(@text) - 2
BEGIN
INSERT INTO @result (Trigram)
VALUES (SUBSTRING(@text, @i, 3));
SET @i = @i + 1;
END
RETURN;
END
Step 3: Insert trigrams from content
INSERT INTO Trigrams (Id, Trigram)
SELECT d.Id, Trigram
FROM Downloads d
CROSS APPLY dbo.GetTrigrams(d.Title);
Step 4: Find similar titles
DECLARE @term NVARCHAR(100) = 'gitar';
DECLARE @trigrams TABLE (Trigram NVARCHAR(10));
INSERT INTO @trigrams
SELECT Trigram FROM dbo.GetTrigrams(@term);
SELECT d.Id, d.Title, COUNT(*) AS Score
FROM Trigrams t
JOIN @trigrams q ON t.Trigram = q.Trigram
JOIN Downloads d ON d.Id = t.Id
GROUP BY d.Id, d.Title
ORDER BY Score DESC;
⚙️ BONUS: Store Suggestions in a Dedicated Table
public class SearchSuggestion
{
public int Id { get; set; }
public string Term { get; set; }
public int PopularityScore { get; set; }
}
You can periodically cache fuzzy hits into this table so that popular typos are returned quickly with autocomplete or “Did you mean?” UI.
🧠 Wrap-Up
Each method serves a different scale:
Method | Best For | Pros |
---|---|---|
FuzzySharp | Shortlists, simple sites | Easy to implement |
ElasticSearch | Enterprise search | Typo-tolerant, scalable |
Trigram in SQL | Medium complexity, fine control | Smart ranking, native SQL |
Want me to build this logic into your DevStack project?
📩 Request access or review my DevStack + pricing to get started.
You can also check out my CV and business skills to understand how I turn search into strategy.