.NET Core 8 Localization That Just Works
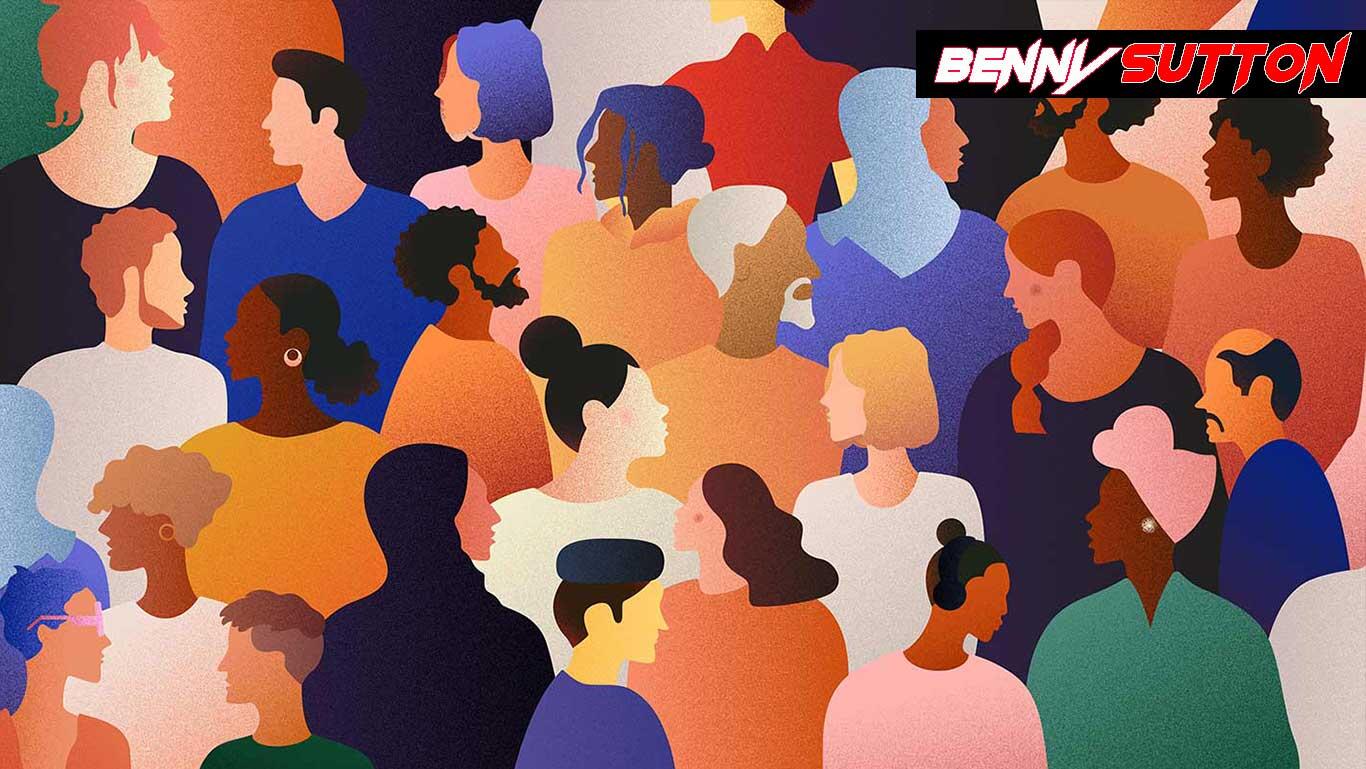
🌐 .NET Core 8 Localization — the easy way
Everyone on the Internet speaks English — unless they don't!
That's a sizeable audience you are missing out on! Billions of potential customers. You might not go all the way to a multi—language site but you will make people feel at home if you show menus, error messages, instructions as a bare minimum.
Adding multilingual support in ASP.NET Core is simple—until it isn’t.
Too many developers hit walls when wiring up .resx
files, configuring supported cultures, or injecting localizers. If you're trying to build a global-ready app in .NET Core 8, here's a minimal, working localization template to start from.
This is the exact pattern I use in production apps from my DevStack. It's fast, elegant, and highly maintainable.
🔹 Folder Structure
ProjectRoot/
├── Resources/
│ ├── SharedResources.resx (Default - English)
│ ├── SharedResources.fr.resx (French)
│ ├── SharedResources.de.resx (German)
│ ├── SharedResources.ru.resx (Russian)
│ ├── SharedResources.zh-CN.resx (Chinese - Simplified)
│ ├── SharedResources.es.resx (Spanish)
🔹 In Program.cs
using MinimalRepro.Localization;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddControllersWithViews();
builder.Services.AddLocalizationServices();
var app = builder.Build();
app.AddLocalizationMiddleware();
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
🔹 Middleware & Services
In Localization/DependencyInjection.cs
:
public static class DependencyInjection
{
public static void AddLocalizationServices(this IServiceCollection services)
{
services.AddLocalization(options => options.ResourcesPath = "Resources");
services.AddMvc()
.AddViewLocalization(LanguageViewLocationExpanderFormat.Suffix)
.AddDataAnnotationsLocalization();
services.Configure<RequestLocalizationOptions>(options =>
{
var supportedCultures = new[] { "en", "ar" };
options.DefaultRequestCulture = new RequestCulture(supportedCultures.First());
options.SetDefaultCulture(supportedCultures.First())
.AddSupportedCultures(supportedCultures)
.AddSupportedUICultures(supportedCultures);
});
}
public static void AddLocalizationMiddleware(this WebApplication app)
{
app.UseRequestLocalization();
}
}
🔹 In Your View
In Views/Index.cshtml
:
@using Microsoft.Extensions.Localization
@inject IStringLocalizer<SharedResources> Localizer
<h1 class="display-4">@Localizer["welcome"]</h1>
Make sure your SharedResources.resx
contains a key "welcome"
.
✅ Why It Matters
- No bloated config
- View + DataAnnotation ready
- Works out of the box with multiple cultures
- Supports Razor Pages or MVC
This pattern is built into my DevStack so you can get it without reinventing the wheel.
💼 Business Case for Localization
If you’re targeting international users, localization isn’t optional—it’s expected.
I’ve spent 30+ years in business helping companies spot global opportunities. One change to their UX, backed by localization, added $25,000 in annual sales.
That’s the kind of insight I bring—not just code, but strategy.
📞 Want Help Going Multilingual?
Whether you're a dev team needing implementation, or a business that wants to grow internationally, I’ve got the tools—and the experience—to make it easy.
Contact me for a localization health check, or review the DevStack for out-of-the-box support.