Which Controller CRUD Actions Should Be Async in ASP.NET Core MVC?
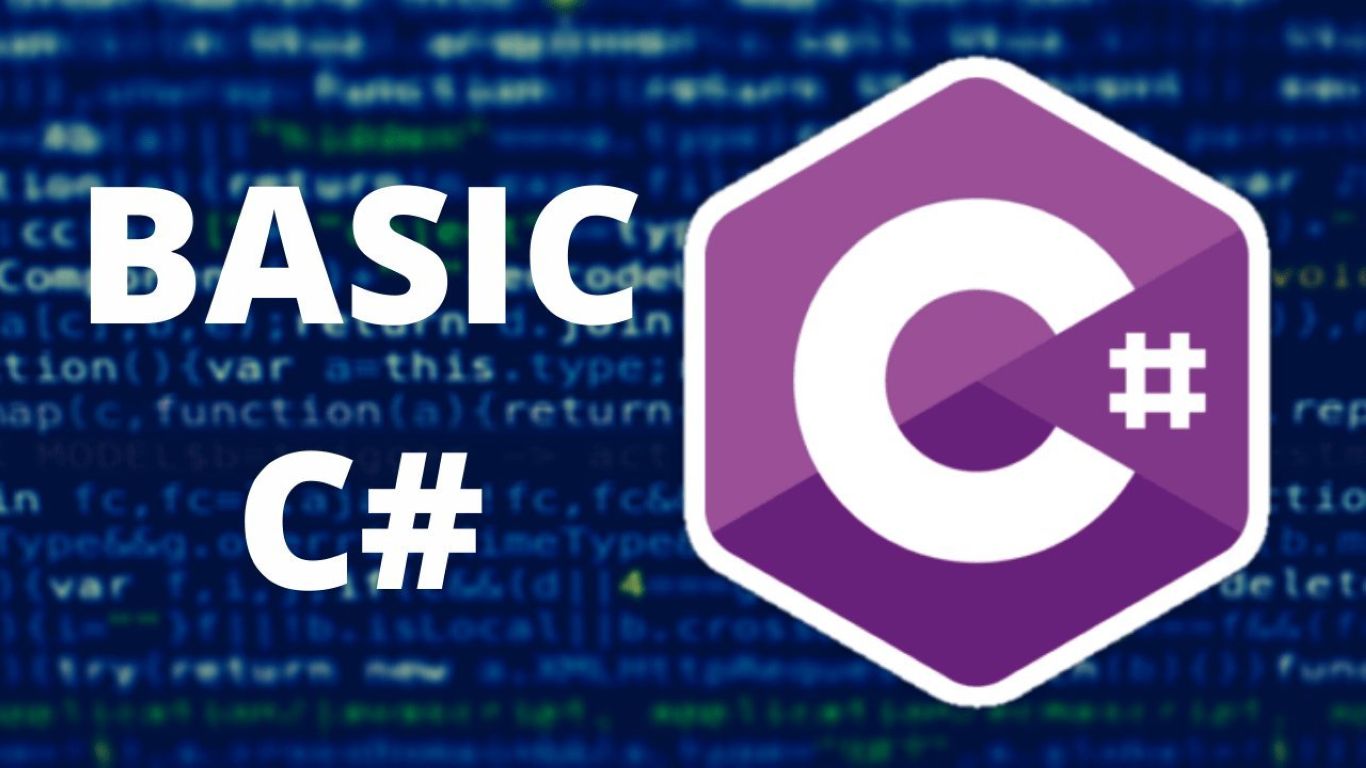
✅ How to Write Clean Async CRUD in ASP.NET Core
If you're building data-driven ASP.NET Core apps, there's a more than a good chance you're performing CRUD operations (Create, Read, Update, Delete). And in 2025, there's no excuse not to make those operations fully asynchronous.
Not only does async make your code more scalable and responsive—it also lays the groundwork for high-performance production apps.
But let’s face it: a lot of async CRUD code online is bloated, repetitive, or... missing the point.
Here’s how I write clean, readable, testable async CRUD in my DevStack—a battle-tested .NET Core 8 codebase that’s powered dozens of production sites.
🔹 Why Async Matters
- ✅ Scalability: Async I/O keeps your threads free while waiting on the database.
- ✅ User Experience: Pages load faster under load because the server isn’t blocked.
- ✅ Future-Proofing: Entity Framework Core is built around async—embrace it.
🔹 Example: Async CRUD in a Razor Pages Model
public class EditModel : PageModel
{
private readonly ApplicationDbContext _context;
public EditModel(ApplicationDbContext context)
{
_context = context;
}
[BindProperty]
public Product Product { get; set; }
public async Task<IActionResult> OnGetAsync(int id)
{
Product = await _context.Products.FindAsync(id);
if (Product == null) return NotFound();
return Page();
}
public async Task<IActionResult> OnPostAsync()
{
if (!ModelState.IsValid) return Page();
_context.Attach(Product).State = EntityState.Modified;
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!await ProductExists(Product.Id)) return NotFound();
throw;
}
return RedirectToPage("Index");
}
private async Task<bool> ProductExists(int id) =>
await _context.Products.AnyAsync(e => e.Id == id);
}
This is lean, fast, and production-grade. And yes—it’s exactly how it’s wired in my DevStack starter kit.
🔹 What Makes This Better?
- Minimal logic in each method
- Async all the way through
- No blocking calls
- Handles not found and concurrency exceptions gracefully
🔹 Want the Whole Thing Done for You?
You could build all this from scratch.
Or…
You could use DevStack, my production-tested starter kit for serious .NET Core projects. It’s built with async-first principles, and includes:
- Fully wired CRUD scaffolding for Razor Pages and Controllers
- Global error handling with Serilog logging
- Localization, SEO, image optimization, and email subsystems out-of-the-box
“I’ve already written the code you need.”
I’m not just a developer—I bring 30+ years of business savvy into every solution. I've built platforms that generate real revenue, not just tick technical boxes.
🧠 Ask me how one feature added $25K/year in recurring sales.
🎯 Ready to Build Smarter?
Let’s chat about your project, your goals, and how DevStack can get you there faster.
→ Request access to DevStack or check out my full tech stack
💬 Want to see more?
You can learn about my consulting services, business insight, and real-world SEO chops at: