How to Monitor Content Security Policy (CSP) Violations in .NET Core 8
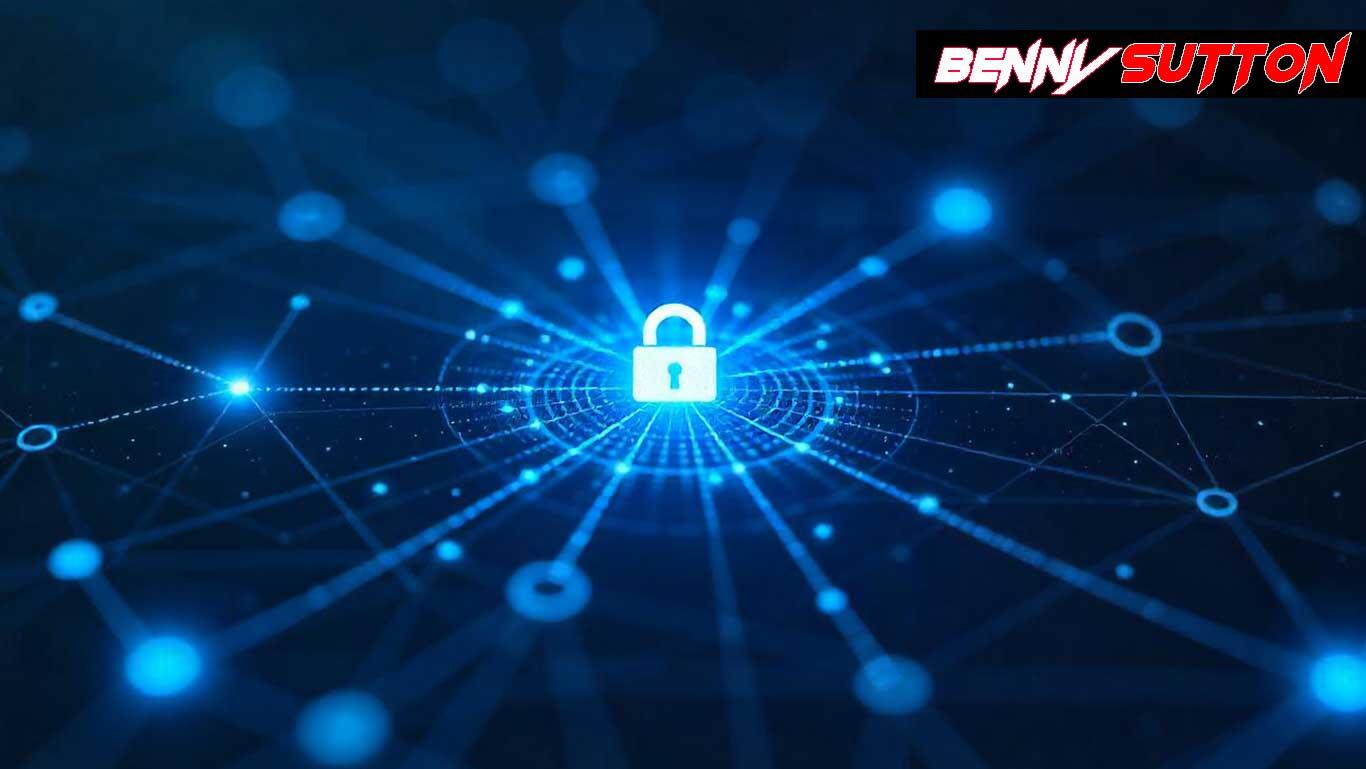
If you're serious about website security, Content Security Policy (CSP) is one of your best defenses against XSS, data injection, and rogue scripts. But how do you know when something's being blocked?
That’s where CSP reporting comes in.
What Is CSP Reporting?
Modern browsers can send you a report whenever they block something due to CSP violations.
For example:
- Inline scripts that violate your policy
- Images or fonts from untrusted sources
- Cross-site script injection attempts
You’ll get a JSON report that you can log, inspect, or even notify on.
🔹 Two Ways to Get CSP Reports
✅ report-uri
(Legacy, widely supported)
Content-Security-Policy: default-src 'self'; report-uri /csp-report
Browsers send a POST to /csp-report
with violation data. Still supported, but deprecated.
✅ report-to
(Modern)
Content-Security-Policy: default-src 'self'; report-to csp-endpoint;
Report-To: {
"group": "csp-endpoint",
"max_age": 10886400,
"endpoints": [{ "url": "https://yoursite.com/csp-report" }],
"include_subdomains": true
}
This is the newer spec — more flexible and works across subdomains. Chrome and Edge support it; Firefox support is partial.
🛠 How to Handle Reports in ASP.NET Core 8
Here’s how I handle it in my DevStack codebase:
1. Add a POST endpoint:
app.MapPost("/csp-report", async context =>
{
using var reader = new StreamReader(context.Request.Body);
var body = await reader.ReadToEndAsync();
Console.WriteLine("🔐 CSP Violation Report:\n" + body);
context.Response.StatusCode = 204;
});
2. Add headers to your middleware:
// Inside your response middleware
response.Headers.Append("Content-Security-Policy", "default-src 'self'; report-to csp-endpoint;");
response.Headers.Append("Report-To", @"{
""group"": ""csp-endpoint"",
""max_age"": 10886400,
""endpoints"": [{""url"": ""https://www.bennysutton.com/csp-report""}],
""include_subdomains"": true
}");
This gives you real-time visibility into what your site is blocking.
🧰 Already Implemented in DevStack
Want to skip the setup?
I’ve already wired this up in my battle-tested .NET Core 8 DevStack — along with CSP headers, SEO metadata, and custom logging hooks.
I can help you:
- ✅ Set up reporting endpoints
- ✅ Store reports in a database
- ✅ Get email alerts on policy violations
Why It Matters to Business
With 30 years of business experience, I don’t just secure websites—I protect customer trust and brand reputation.
Ask me how I hardened a legacy web app with CSP and flagged 40+ third-party script leaks—within a week of going live.
You can review my background in tech and business leadership, or request a consult if you’re ready to level up your site security.
CTA
Ready to stop guessing and start logging security violations?