Getting Validation Right in ASP.NET Core
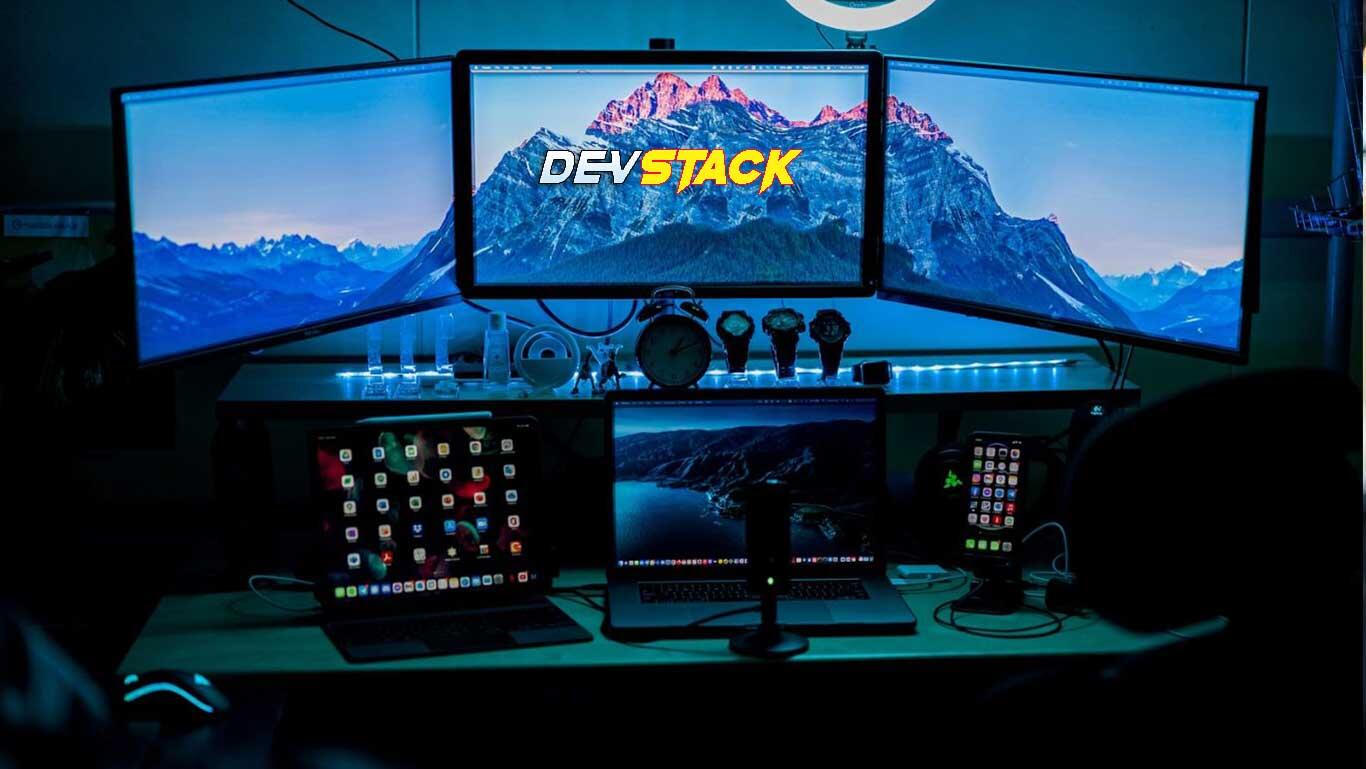
When Front-End Meets Back-End: Getting Validation Right in ASP.NET Core
Form validation isn't just a checkbox—it’s a critical intersection of UX, security, and data integrity.
It’s also where front-end meets back-end—and where things often go wrong.
Whether you're using ASP.NET Core with data annotations, or injecting dynamic content like a custom RTE, validation needs to be robust and flexible.
“I remember spending a whole day untangling conflicting validation logic in a hybrid Razor + jQuery form. I wish someone had just built it right the first time.”
✅ The Modern Setup: ASP.NET + jQuery Validation (Without Conflicts)
The trick is to layer unobtrusive validation with jQuery rules, without overwriting one another.
Here's how I’ve done it in DevStack:
🔹 1. Enable Unobtrusive Validation
Include these scripts in your layout:
<script src="~/lib/jquery/dist/jquery.min.js"></script>
<script src="~/lib/jquery-validation/dist/jquery.validate.min.js"></script>
<script src="~/lib/jquery-validation-unobtrusive/jquery.validate.unobtrusive.min.js"></script>
🔹 2. Use Razor Helpers for Data Binding
Let ASP.NET generate the proper data-val-*
attributes:
@Html.TextAreaFor(m => m.Description, new {
@class = "form-control", rows = 10
})
@Html.ValidationMessageFor(m => m.Description, "", new { @class = "text-danger" })
In your model:
[Required(ErrorMessage = "Description is required")]
[StringLength(4000, ErrorMessage = "Too long")]
public string Description { get; set; }
🔹 3. Enhance with Custom jQuery Rules
You can add dynamic checks like word count:
$(function () {
const form = $("#myForm");
$.validator.unobtrusive.parse(form);
$.validator.addMethod("wordcount", function (value, element, param) {
return value.trim().split(/\s+/).length <= param;
}, "Too many words!");
$("textarea[name='Description']").rules("add", {
wordcount: 200
});
});
This preserves your back-end rules while allowing for enhanced front-end logic.
🔹 Why Validation Matters
Validation isn't just about showing red warnings:
- ✅ It protects your database from bad input.
- ✅ It improves conversion rates with better UX.
- ✅ It gives users confidence in your platform.
- ✅ It guards against malicious scripts and misuse.
"Most bugs I debugged for clients started with bad or inconsistent validation. That's why DevStack builds it in from both sides—from the database to the DOM."
🚀 Want It Done Right the First Time?
I’ve already written this code. It’s in DevStack, my battle-tested .NET Core Core 8 platform—ready for projects that need:
- Secure, unobtrusive validation
- Rich forms with dynamic fields
- Full server+client integration (no JS spaghetti)
You can also check my background in tech and business. I build apps that work—and work for you.
✅ TL;DR
What You Need | Why It Matters |
---|---|
Unobtrusive + jQuery validation | Best of both worlds |
Razor + annotations | Easier to maintain |
Custom JS rules | UX flexibility |
DevStack boilerplate | Saves hours of dev time |
📩 Ready to Stop Fighting Forms?
Let’s validate something together—like your next project.
→ Request access to DevStack or Book a quick demo