Syntax Highlighting with Prism.js in .NET Projects
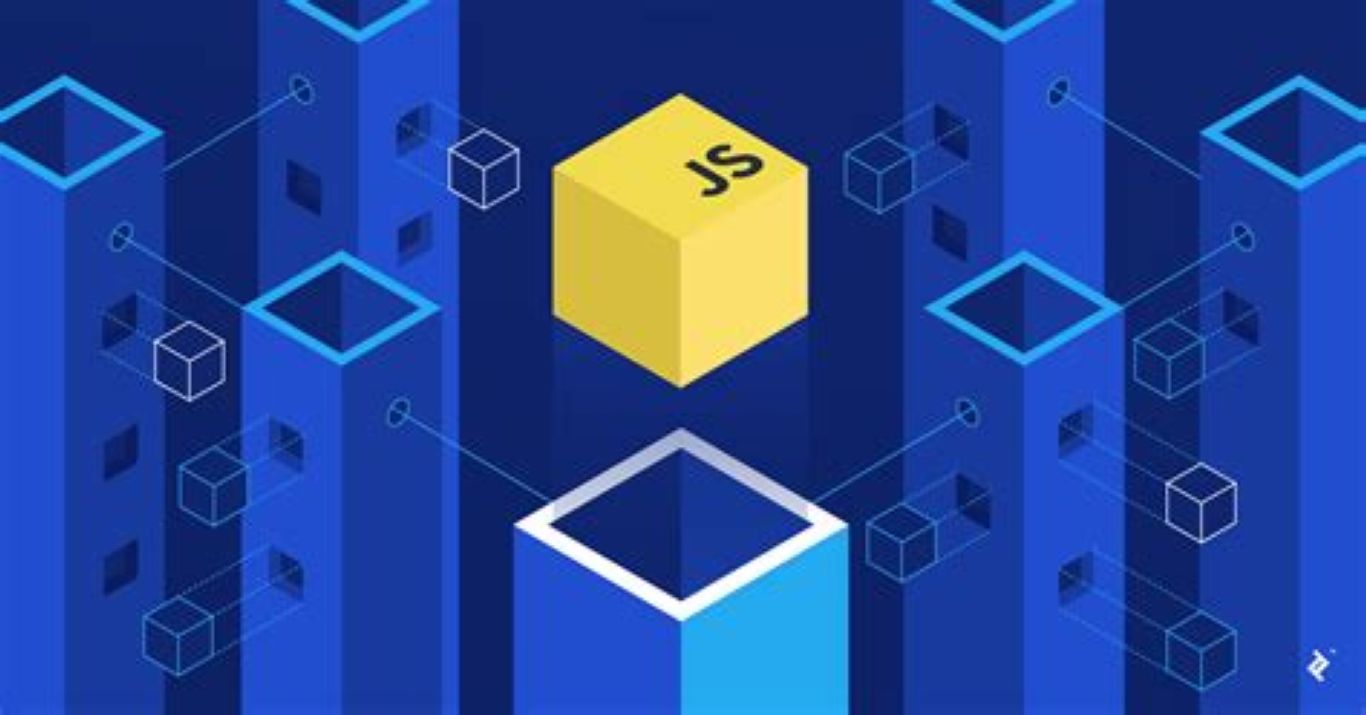
If you're building a technical site or developer documentation, you already know how important clean, readable code examples are. But integrating syntax highlighting (especially across multiple languages with proper formatting and copy buttons) isn’t always plug-and-play.
DevStack includes a full Prism.js setup out of the box — pre-wired, styled, and tested for .NET developers.
✅ Why Prism.js?
Prism.js is a lightweight, extensible syntax highlighter that works well with modern frontend frameworks. Unlike heavier libraries, Prism lets you:
- Load only the languages you need
- Add plugins like line numbers, autolinker, or copy to clipboard
- Style with your own CSS or use one of many ready-made themes
💡 What It Looks Like (Live)
using System;
using System.IO;
class Program
{
static void Main()
{
string path = "example.txt";
if (File.Exists(path))
{
Console.WriteLine("Found file: " + path);
}
}
}
The above snippet is fully styled using Prism.js with support for:
- ✅ Language detection via class (e.g.,
language-csharp
) - ✅ Optional line numbers
- ✅ Copy button tooltip
🔧 How to Set It Up
Here’s what you need to make Prism work on your own project:
1. Include CSS & JS
In <head>
:
<link href="https://cdnjs.cloudflare.com/ajax/libs/prism/1.29.0/themes/prism.min.css" rel="stylesheet" />
<link href="https://cdnjs.cloudflare.com/ajax/libs/prism/1.29.0/plugins/line-numbers/prism-line-numbers.min.css" rel="stylesheet" />
Before `