Web Workers vs. Service Workers made easy
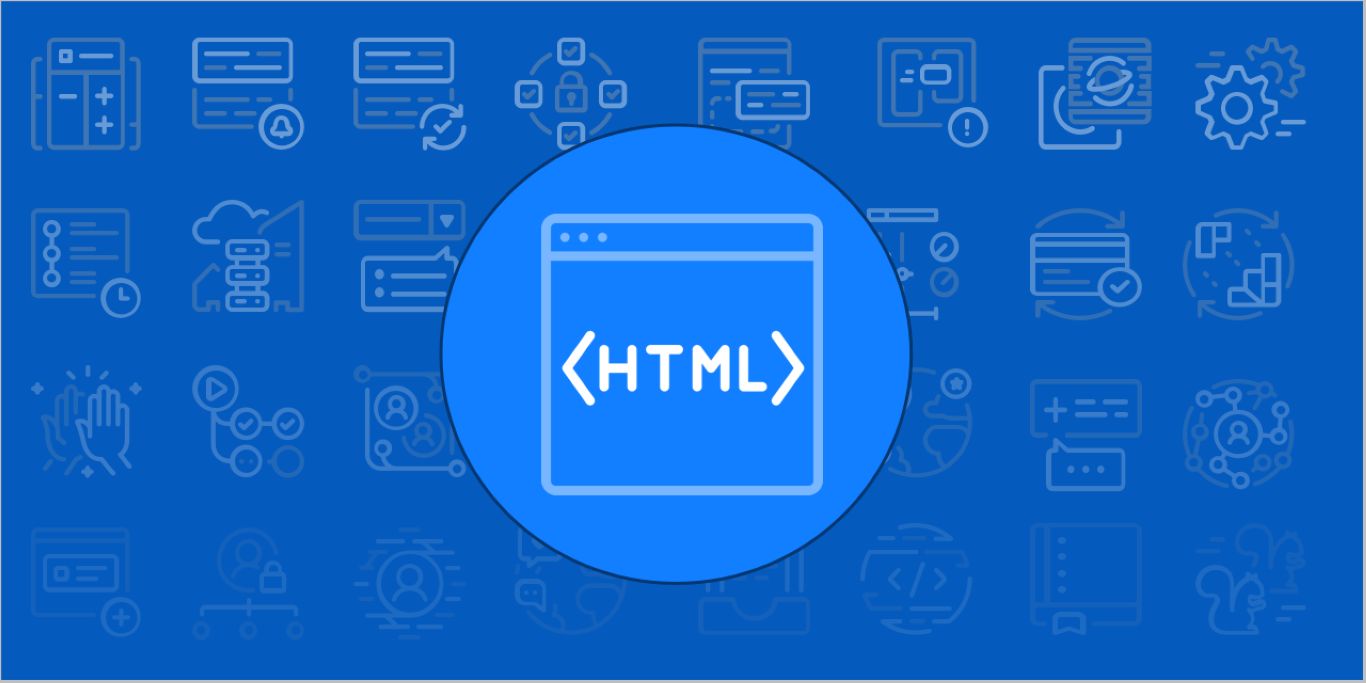
Web Workers vs. Service Workers: A Full-Stack Dev’s Secret Weapons!
Modern web apps need to feel snappy, responsive, and resilient—especially when you're dealing with rich media like audio, images, or offline content. That’s where Web Workers and Service Workers shine.
These powerful browser APIs allow you to offload work from the main thread, dramatically improving user experience. But they do very different things, and knowing when to use each is key.
🔹 Web Workers – Run Complex Logic Without Freezing the UI
Web Workers are your go-to for CPU-intensive tasks—things like audio analysis, image processing, or heavy number crunching. They're perfect when you want to keep your UI buttery-smooth.
⛔ No DOM access — these run in a separate thread.
Use Web Workers when you need to:
Task | Why |
---|---|
Audio analysis | Keeps music playback smooth |
Image manipulation | Applies filters without UI lag |
Markdown rendering | Parses large text in background |
Crypto operations | Hashes data without blocking |
Data crunching | Handles long loops, stats, etc. |
Example:
// worker.js
onmessage = function (e) {
const result = heavyComputation(e.data);
postMessage(result);
};
// main.js
const worker = new Worker("worker.js");
worker.postMessage(data);
worker.onmessage = (e) => updateUI(e.data);
This kind of thread-splitting architecture is already baked into DevStack, my full-stack .NET Core platform for performance-minded builders.
📦 Service Workers – Power Offline Support and Caching
Service Workers sit between your site and the network—essentially a programmable network proxy. They're ideal for:
- Offline support
- Caching assets
- Background sync
- Push notifications
Use Service Workers to:
Feature | Why |
---|---|
Offline fallback | Let users continue even if disconnected |
Smart caching | Instantly load assets from cache |
Background sync | Retry failed uploads or form posts |
Push notifications | Re-engage users with timely alerts |
Example:
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/sw.js');
}
In DevStack, you can scaffold a production-ready Service Worker to cache routes, fallback pages, and even images for media-heavy platforms.
💡 TL;DR — Which Worker for Which Job?
Worker Type | Best For | Runs In |
---|---|---|
Web Worker | CPU-heavy, background logic | Separate thread |
Service Worker | Offline, caching, background sync | Browser proxy layer |
Why This Matters for You
If you're building media-rich experiences—MP3 players, Markdown editors, interactive canvases—you need non-blocking logic.
If you're building apps users expect to work offline or load fast on spotty mobile networks, you need smart caching and background sync.
I’ve already built this logic into DevStack. You can grab it, use it, or ask me to implement it for your use case.
Want to see Web Workers live-parsing audio files? Or Service Workers powering an offline song builder?
Visit my CV, explore DevStack, or check out my business offering to see how I can help.
CTA
If you want a head start with proven architecture—and the business insight to monetize what you're building—let’s talk. I’ll help you ship faster and smarter, not just code deeper.