Routes in ASP.NET MVC
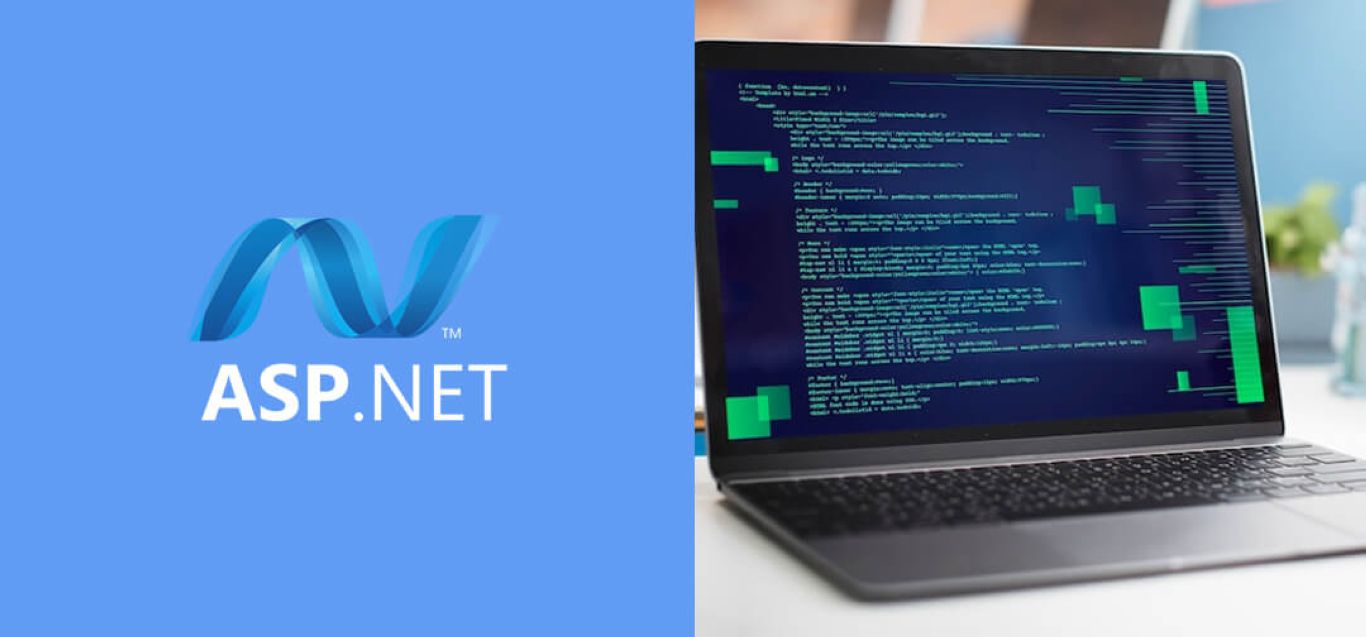
🔀 Understanding Routes in ASP.NET & ASP.NET Core
Routing determines how your app responds to incoming URLs.
With DevStack, a flexible routing engine is already wired in — so you can focus on features, not boilerplate.
🚧 Classic MVC Routing (ASP.NET Framework)
In traditional MVC projects, routes are registered in the RouteConfig.cs
file under App_Start
.
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }
);
}
}
➕ Adding Custom Routes
You can define additional routes above the default one to handle specific URL patterns:
routes.MapRoute(
name: "Product",
url: "Product/{id}",
defaults: new { controller = "Product", action = "Details" },
constraints: new { id = @"\d+" } // Only match numeric IDs
);
✅ Already handled in DevStack — routes are automatically prioritized by specificity.
📄 Razor Pages Routing
For Razor Pages in ASP.NET Core, you define routes using the @page
directive at the top of .cshtml
files.
@page "/product/{id}"
@model ProductModel
<h1>Product ID: @Model.Id</h1>
Route Binding in PageModel:
[BindProperty(SupportsGet = true)]
public int Id { get; set; }
🎯 This enables GET-friendly model binding — ideal for search, filters, and SEO-friendly detail pages.
🎯 Attribute Routing in ASP.NET Core
Attribute routing gives you controller-level or action-level route definitions:
[Route("api/[controller]")]
public class ProductsController : Controller
{
[HttpGet("{id}")]
public IActionResult GetProduct(int id)
{
return Ok(new { ProductId = id });
}
}
✅ DevStack fully supports attribute routing, fallback routes, and route constraints like regex and enums.
🧪 Testing Routes
- Manual: Hit your routes in the browser or via Postman.
- Automated: Unit tests can verify route-to-action resolution.
- Debugging:
- Use Routing Debugger for ASP.NET
- Or enable endpoint routing logs in ASP.NET Core:
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
💡 TL;DR: Routing Made Easy
Feature | Classic MVC | ASP.NET Core | DevStack Adds |
---|---|---|---|
Convention-based routing | ✅ | ✅ | ✅ With layered fallback and priorities |
Attribute routing | ❌ | ✅ | ✅ Already baked in |
Razor Page routes | ❌ | ✅ | ✅ Fully supported |
Regex/constraint support | ✅ | ✅ | ✅ With smart defaults |
Route debugging tools | Limited | Good via logging | ✅ Verbose route tracing helper |
🚀 Skip the Setup — It’s Already Done
Every route type mentioned above is already supported in my DevStack codebase. You get:
- ✅ Pre-wired routes for MVC, Razor Pages, APIs
- ✅ Attribute routing and slugs
- ✅ Clean SEO-friendly URLs
- ✅ Route helpers and fallback logic
If you’re building anything from a blog to a SaaS app and want to skip the plumbing — I’ve already written the code you need.
Let me help you fast-track your .NET Core project — with proper routing, SEO, and structure from day one.