Migrating ASP.NET Identity from MVC 4 to Core 8
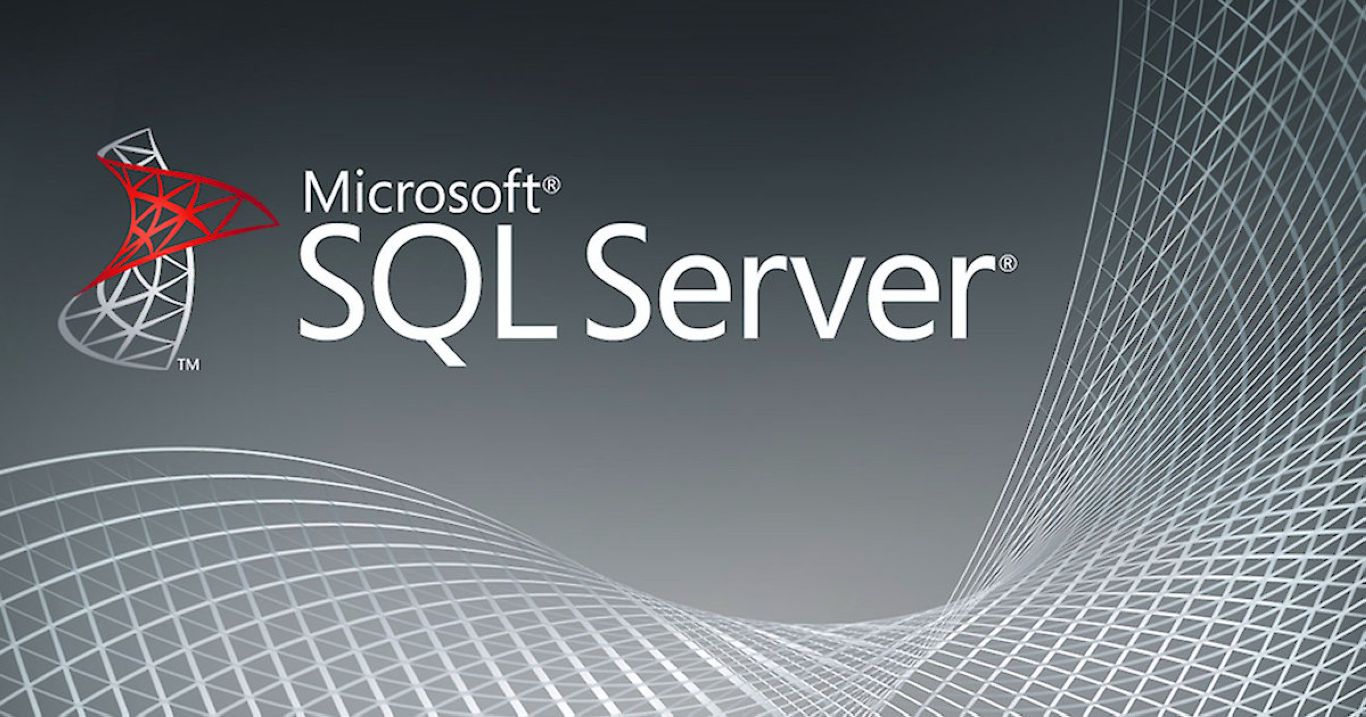
🔄 Modernizing a legacy MVC 4 project to ASP.NET Core Core 8 is no small feat—especially when Identity is involved. If you’ve been through it, you already know. If you haven’t… you're in for a surprise.
I’ve done it, and I’ll be honest: it’s messy.
I’ve worked on every version of ASP—from Classic ASP to Core Core 8.
Back in 1998, Microsoft gave me early beta access on 3½" floppies!
So yes, I’ve seen every twist in the ASP.NET journey—and I now offer a service to migrate your app cleanly from Identity 4 to Core Core 8 without the pain.
❌ Why It’s Not Plug-and-Play
Migrating Identity means rewriting assumptions baked into your user management. You’ll likely need to:
- Drop and re-create role and user tables
- Rebuild relationships manually
- Align with the non-nullable, async-first architecture of Core
- Avoid cluttering
Program.cs
with admin seed logic
If you’ve tried doing it with just Add-Migration
and Update-Database
, you already know: that’s the easy part.
✅ Two Smart Ways to Seed Identity Roles
Here’s what worked for me:
🔹 SQL Approach (Clean and Manual)
-- Create Admin Role
INSERT INTO AspNetRoles (Id, Name, NormalizedName)
VALUES (NEWID(), 'Admin', 'ADMIN');
-- Find your UserId
SELECT Id FROM AspNetUsers WHERE Email = '[email protected]';
-- Assign Admin Role
INSERT INTO AspNetUserRoles (UserId, RoleId)
VALUES ('your-user-id', (SELECT Id FROM AspNetRoles WHERE Name = 'Admin'));
🔹 Admin Controller Approach (Reusable and Safe)
Create an AdminController
to assign roles without bloating Program.cs
.
[Authorize(Roles = "Admin")]
public class AdminController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<BenIdentityUser> _userManager;
public AdminController(RoleManager<IdentityRole> roleManager, UserManager<BenIdentityUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
[HttpPost]
public async Task<IActionResult> CreateRole(string roleName)
{
if (!await _roleManager.RoleExistsAsync(roleName))
await _roleManager.CreateAsync(new IdentityRole(roleName));
return RedirectToAction("Index");
}
[HttpPost]
public async Task<IActionResult> AssignRole(string email, string roleName)
{
var user = await _userManager.FindByEmailAsync(email);
if (user != null && !await _userManager.IsInRoleAsync(user, roleName))
await _userManager.AddToRoleAsync(user, roleName);
return RedirectToAction("Index");
}
}
Use a basic Razor form to let you manage roles safely via browser.
🛠️ DevStack Includes Identity Built for Core Core 8
The version of Identity that ships with DevStack is already:
- Async from the ground up
- Integrated with a custom user model (
BenIdentityUser
) - runs on its own Db with a seperate connection for modularity
- Designed for flexible role and claim management
- Easily extensible with email confirmation, 2FA, external logins, and more
No surprises. Just working code.
🤝 Done-For-You Migration Services
You could spend days figuring this out yourself, like I did (seriously—this took a whole day 😩).
Or you could bring me in.
- View my CV for 40+ years of hands-on development and architecture experience
- Explore my DevStack to see the boilerplate you’ll inherit
- Read more about my business-first consulting if you want results, not just code
Let me handle the migration so you can focus on your users and business—not Identity plumbing.
📩 Ready to Migrate?
Let’s move you from legacy to launch-ready.
Contact me for a quick chat about your Identity upgrade.
`