Handling Post-Login Redirects in ASP.NET Core
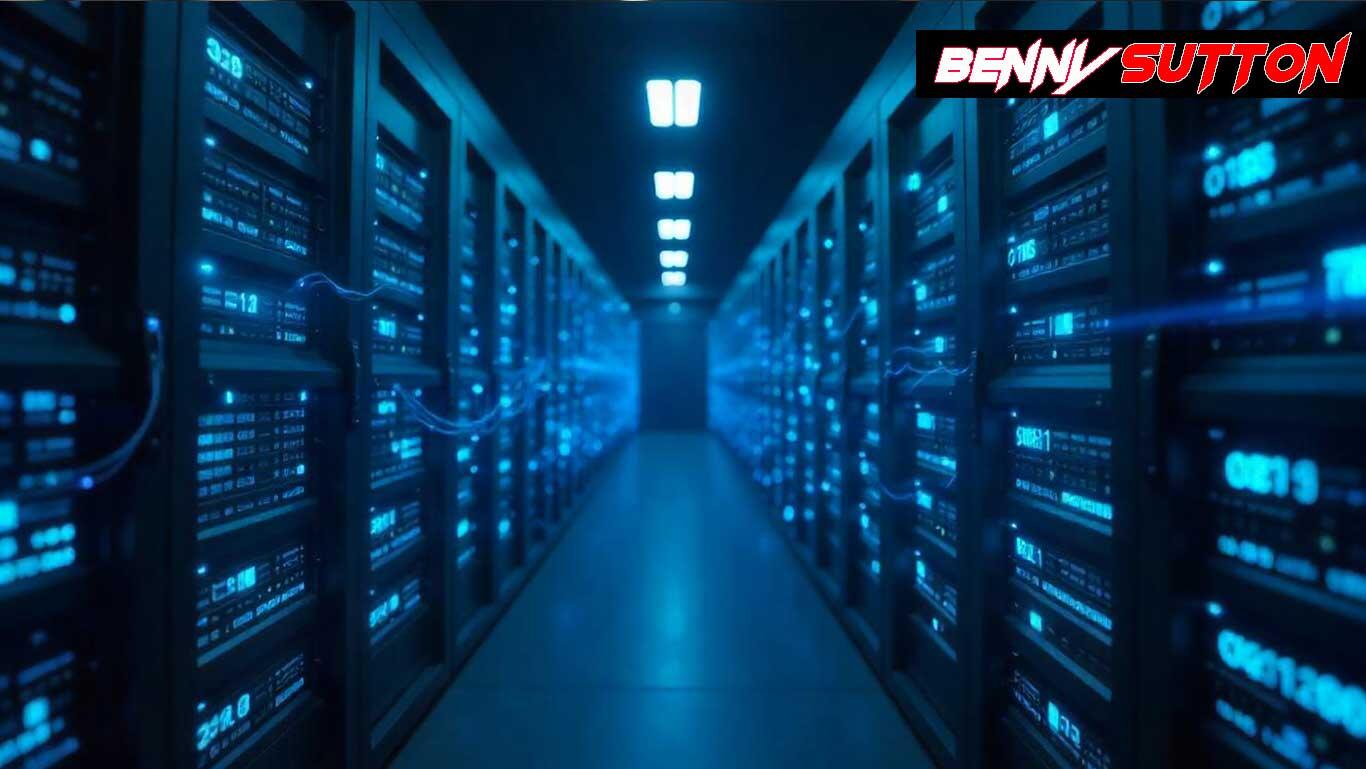
One of the most common pain-points for web developers is how to return a user to the page they originally requested after logging in. Fortunately, ASP.NET Core handles this cleanly—and securely—if you know how to wire it up properly.
If you're building secure user experiences, especially in a multi-page app or dashboard, this pattern is essential.
The Core Concept: ReturnUrl
When a user tries to visit a protected page like /Dashboard
and isn't authenticated, the framework automatically redirects them to:
/Account/Login?ReturnUrl=%2FDashboard
After a successful login, you should redirect them back to this ReturnUrl
.
🔧 Step-by-Step Setup
1. Protect Your Page with [Authorize]
[Authorize]
public IActionResult Dashboard()
{
return View();
}
2. Capture the ReturnUrl in the Login Actions
GET:
public IActionResult Login(string returnUrl = null)
{
ViewBag.ReturnUrl = returnUrl;
return View();
}
POST:
[HttpPost]
public async Task<IActionResult> Login(LoginViewModel model, string returnUrl = null)
{
if (!ModelState.IsValid) return View(model);
var result = await _signInManager.PasswordSignInAsync(model.Email, model.Password, model.RememberMe, lockoutOnFailure: false);
if (result.Succeeded)
{
if (!string.IsNullOrEmpty(returnUrl) && Url.IsLocalUrl(returnUrl))
{
return Redirect(returnUrl); // ✅ Secure redirect
}
return RedirectToAction("Index", "Home");
}
ModelState.AddModelError("", "Invalid login attempt.");
return View(model);
}
🔐 Important: Validate the ReturnUrl
Never redirect blindly. Url.IsLocalUrl()
protects you from open redirect vulnerabilities (which can be exploited in phishing attacks).
🧠 Preserve ReturnUrl in the Login Form
Don’t forget to echo the ReturnUrl
into your login form as a hidden input:
<form asp-action="Login">
<input type="hidden" name="returnUrl" value="@ViewBag.ReturnUrl" />
<!-- Other fields like Email, Password, etc. -->
</form>
💡 Want to Always Redirect to a Dashboard?
If no ReturnUrl
exists (e.g., user logs in directly), you can add a fallback redirect to /Dashboard
or any default landing page. This is part of the custom logic built into my DevStack platform.
This post-login redirect pattern is just one small part of a broader user management system I’ve built into DevStack.
I’ve spent years solving real-world user workflows, so you don’t have to.
Want to see how I’d integrate this into your site or improve your existing identity setup?
Check out my CV and business background—I blend technical depth with decades of commercial insight.
✅ Summary
- Use
[Authorize]
to trigger login redirects automatically. - Capture
ReturnUrl
in both GET and POST login actions. - Validate the redirect with
Url.IsLocalUrl()
. - Echo it back in a hidden form field.
You can copy this and be up and running in minutes—or if you'd prefer someone who’s done it many times before, get in touch.
Sometimes it’s faster (and cheaper) to have someone who already wrote the code.