c# User cannot convert Environment.NewLine to char error
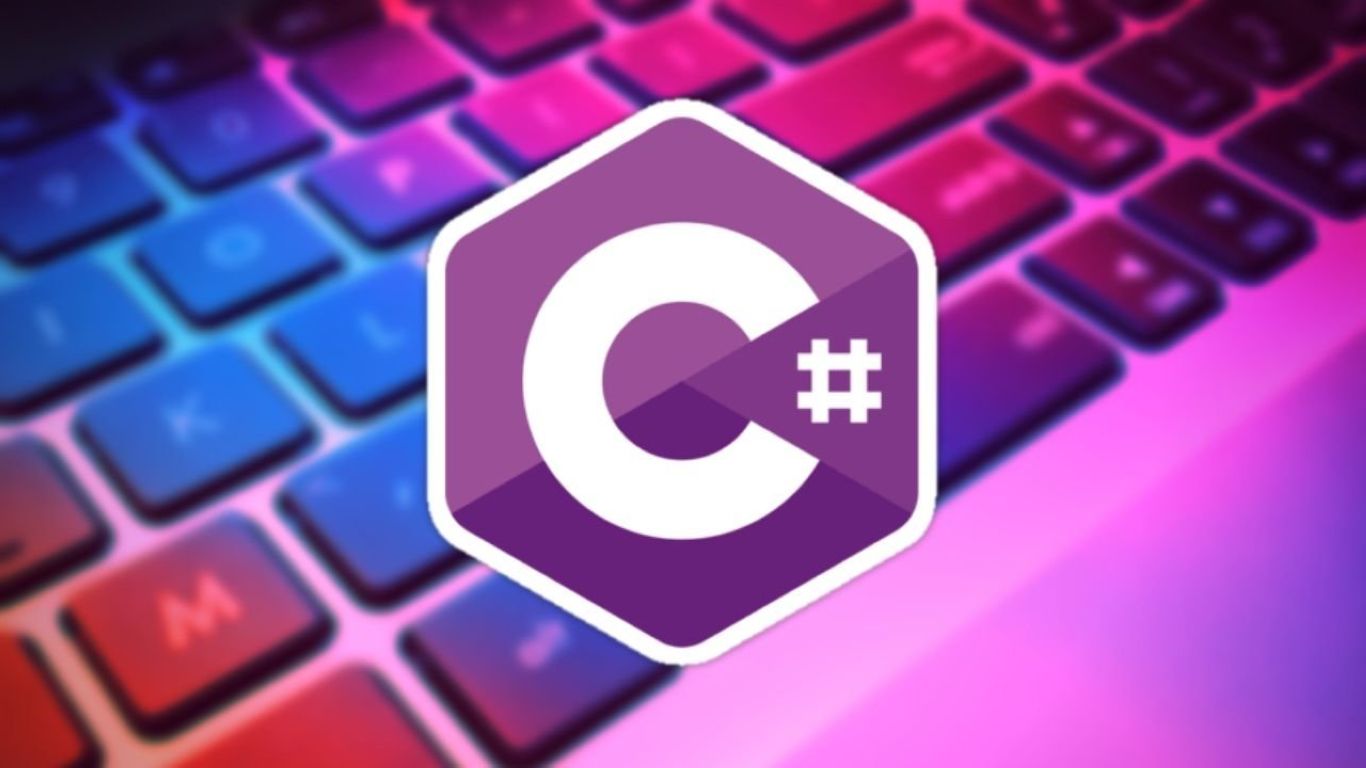
🧵 How to Split a String by New Lines in C#
(And Why Environment.NewLine
Doesn’t Always Work)
If you’ve tried splitting a string using Environment.NewLine
, you’ve probably hit an unexpected snag: it doesn’t behave the way you’d expect, especially across platforms or when working with dynamic input.
Let’s break it down—and more importantly, let me show you the bulletproof way I’ve already implemented in my own DevStack.
❌ What Doesn’t Work
// This won't compile or behave as expected
string[] lines = sInput.Split(Environment.NewLine);
Why? Because:
Environment.NewLine
is a string, butSplit(string)
matches exact strings, not character sequences.- It fails when input uses different newline styles (
\n
,\r
, or\r\n
)—which is common when data comes from user input, APIs, or cross-platform files.
✅ The Correct Way (Cross-Platform Safe)
To properly split text on any newline, use:
string[] lines = sInput.Split(new[] { '\n', '\r' }, StringSplitOptions.RemoveEmptyEntries);
🔍 This safely handles:
\n
(Unix/Linux/macOS)\r\n
(Windows)\r
(Classic Mac)
And skips blank lines using RemoveEmptyEntries
.
🧪 Real Example: Wrapping Untagged Paragraphs
Suppose you want to process a user-submitted block of text, wrap each paragraph in <p>
tags, but only if the line doesn't already start with HTML:
using System;
using System.Collections.Generic;
using System.Text;
class Program
{
static void Main()
{
string sInput = "This is a paragraph.\nThis is another paragraph.\n\nThis is a third paragraph.";
IEnumerable<string> paragraphs = sInput.Split(
new[] { '\n', '\r' },
StringSplitOptions.RemoveEmptyEntries
);
StringBuilder result = new StringBuilder();
foreach (string paragraph in paragraphs)
{
if (paragraph.StartsWith("<")) // already tagged
{
result.AppendLine(paragraph);
}
else
{
result.AppendLine("<p>" + paragraph + "</p>");
}
}
Console.WriteLine(result.ToString());
}
}
💡 Bonus: Split by Paragraph (Double Newlines)
Want to break by actual paragraphs instead of single lines?
string[] paragraphs = Regex.Split(sInput, @"\r?\n\r?\n");
This is ideal for blog editors, comment systems, or Markdown processing—already baked into my rich text editor module.
🚀 Already Built for You
If you're building a CMS, editor, or content parser in ASP.NET Core, this kind of utility is just one of hundreds of real-world, developer-ready features in my DevStack codebase.
Why reinvent the wheel when I’ve already done the hard work?
📄 Read my CV — and let’s work together.