Add Star Ratings to Your .NET Website with JSON-LD
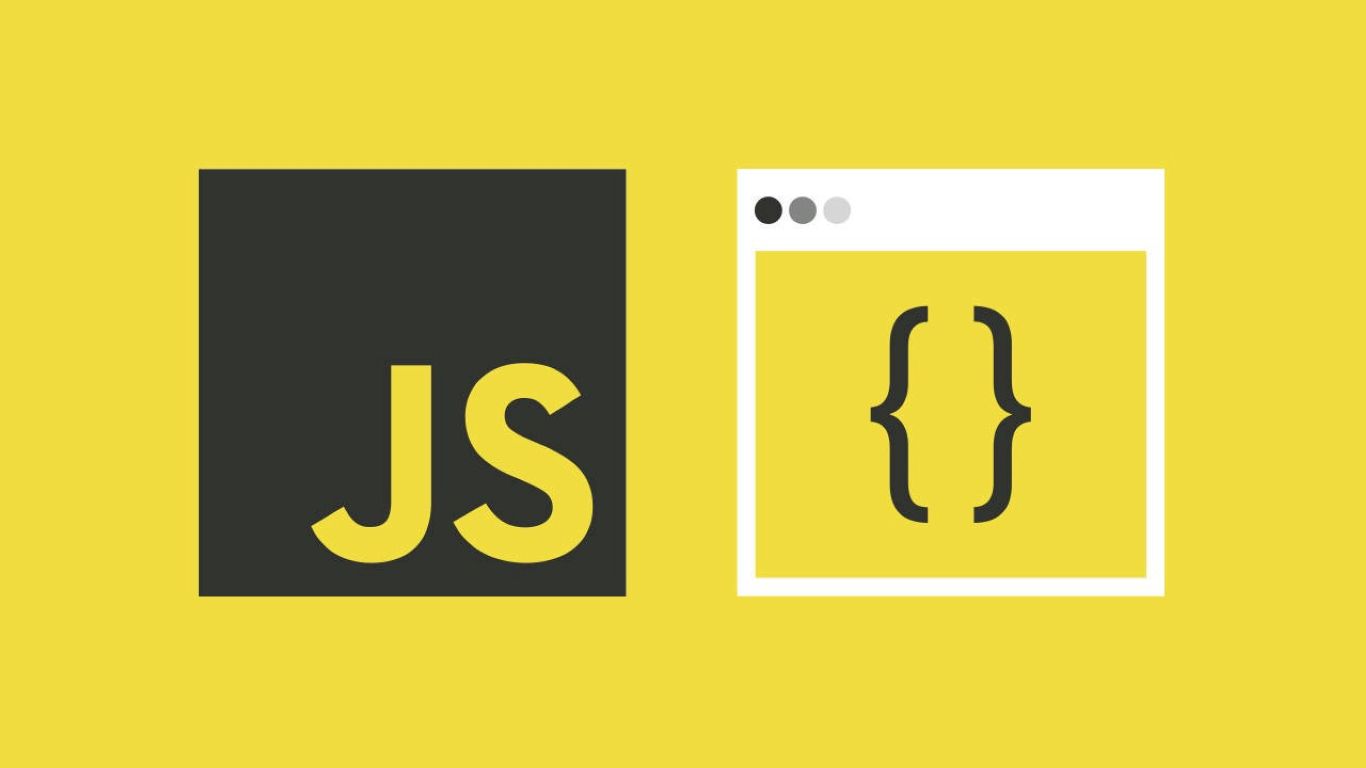
If you’re building a music, download, or content platform with ASP.NET Core, adding structured star ratings (via JSON-LD) helps your content stand out in search results. Not only do ratings improve your credibility — they boost visibility and click-through rate (CTR) on Google.
Here’s how to do it properly, and why you might want a production-ready solution that saves time.
🔹 What Are Star Ratings in Search Results?
Star ratings are part of Google Rich Results. When you add the correct Schema.org AggregateRating
to your JSON-LD, Google can display ⭐️ directly in the SERPs.
This makes your listing more eye-catching and trustworthy, especially for:
- Music tracks or albums
- File downloads
- Blog posts or tutorials
- Product pages
- Educational content
✅ Step-by-Step: Add AggregateRating
to JSON-LD in ASP.NET Core
1️⃣ Update Your Download
Model
public class Download
{
public int DownloadID { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public string Slug { get; set; }
public DateTime DateCreated { get; set; }
public string Genre { get; set; }
public string Author { get; set; }
public string RelatedPage { get; set; }
// Add rating properties
public int RatingCount { get; set; } = 0;
public double RatingValue { get; set; } = 0.0;
}
2️⃣ Update Your GetJsonLd()
Method
Include this logic in your Details
action or view component:
private string GetJsonLd(Download download)
{
var jsonLd = new Dictionary<string, object>
{
["@context"] = "https://schema.org",
["@type"] = "MusicRecording",
["name"] = download.Name,
["description"] = download.Description,
["url"] = GetCanonicalUrl(id: download.DownloadID, slug: download.Slug),
["datePublished"] = download.DateCreated.ToString("yyyy-MM-dd"),
["genre"] = download.Genre,
["author"] = new Dictionary<string, string>
{
["@type"] = "Person",
["name"] = download.Author
},
["inAlbum"] = new Dictionary<string, string>
{
["@type"] = "MusicAlbum",
["name"] = download.RelatedPage
}
};
if (download.RatingCount > 0)
{
jsonLd["aggregateRating"] = new Dictionary<string, object>
{
["@type"] = "AggregateRating",
["ratingValue"] = Math.Clamp(download.RatingValue, 1.0, 5.0),
["ratingCount"] = download.RatingCount,
["bestRating"] = 5,
["worstRating"] = 1
};
}
return JsonConvert.SerializeObject(jsonLd);
}
📌 Example Output
{
"@context": "https://schema.org",
"@type": "MusicRecording",
"name": "Amazing Song",
"description": "This is a fantastic jazz track.",
"url": "https://bennysutton.com/Downloads/123-amazing-song",
"datePublished": "2024-02-01",
"genre": "Jazz",
"author": {
"@type": "Person",
"name": "Benny Sutton"
},
"aggregateRating": {
"@type": "AggregateRating",
"ratingValue": 4.7,
"ratingCount": 5,
"bestRating": 5,
"worstRating": 1
}
}
Google now has everything it needs to render ⭐️ in your snippet.
🔍 Why Ratings Matter
- ✅ Improve CTR by making your pages more clickable
- ✅ Establish trust and authority
- ✅ Surface higher in Google Image or Music results
- ✅ Support SEO without altering visible content
💡 Pro Tip from Experience
It took a lot of trial and error to get this working cleanly with ASP.NET Core, especially without introducing validation issues or bloat.
Now it’s built into my DevStack platform — so you don’t have to wrestle with schema edge cases, SEO flags, or missing data. Just plug it in and go.
🎯 Demo It in Action
Check out this voting page with live ratings — a real-world use case built with the exact logic above.
🧠 Business Tip
You don’t just need code — you need to understand the business value of features like this.
I once added structured metadata to a download catalog that led to a 15% increase in organic traffic — and over $25,000 in additional revenue.
Learn more about how I combine business strategy and .NET execution at my consulting overview.
✅ TL;DR
Feature | Benefit |
---|---|
AggregateRating | Google stars in search results |
ratingCount > 0 | Avoids empty or invalid JSON-LD |
Full integration | Built into DevStack |
SEO-friendly | Passes all schema validators |
Want to skip the hassle and get this done right?
Request access to DevStack or contact me to explore how I can help accelerate your project.