Real-Time Web Made Easy with SignalR and .NET
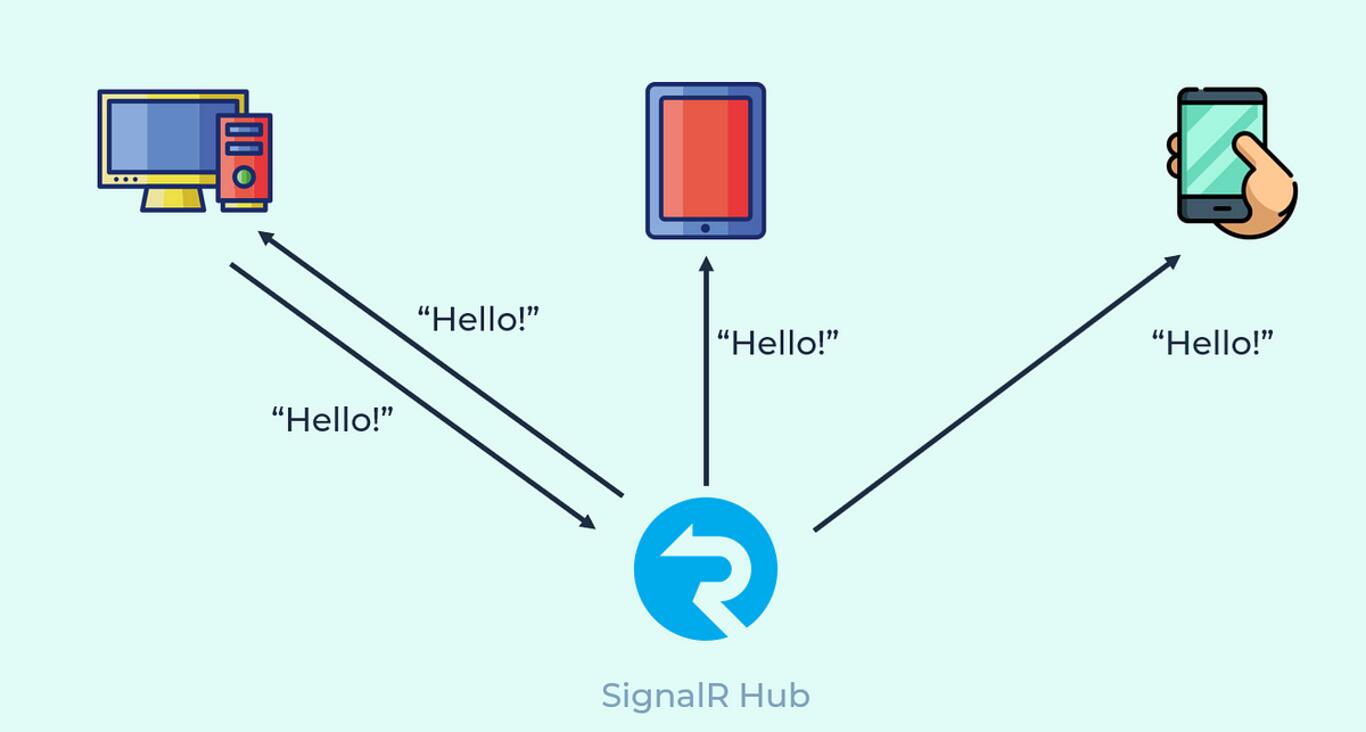
If you've ever wanted to push live updates to users without them refreshing the page—welcome to SignalR. It's built into ASP.NET Core and gives you real-time power with barely any setup.
🔹 What Is SignalR?
SignalR is a real-time communication library for ASP.NET Core (and legacy .NET). It allows server-to-client messaging over WebSockets (with graceful fallback to SSE or long polling).
Think of it as a smart WebSocket wrapper with a clean API and deep .NET integration.
✅ What Can You Do With SignalR?
Use Case | Example |
---|---|
Notifications | Show alerts when a user logs in or uploads a file |
Live dashboards | Display real-time analytics or telemetry |
Chat applications | Enable messaging between users |
Music tools | Sync tempo or chord changes across users |
Multiplayer games | Reflect player moves instantly |
Collaborative editing | Show what others are working on in real time |
🧠 Why SignalR Matters
Without it, your app is constantly polling the server—wasting bandwidth and lagging behind real events. With SignalR, your app stays responsive, modern, and engaging.
It’s already baked into DevStack, my full-stack .NET Core Core 8 boilerplate. So if you want to wire up live alerts, dashboards, or syncing—you're already halfway there.
I’ve already written the code. You just need to plug it in.
⚙️ Minimal SignalR Setup
Server-side (Hub class):
public class SongHub : Hub
{
public async Task BroadcastChord(string chordName)
{
await Clients.All.SendAsync("ReceiveChord", chordName);
}
}
Client-side (JavaScript):
const connection = new signalR.HubConnectionBuilder()
.withUrl("/songHub")
.build();
connection.on("ReceiveChord", chord => {
highlightChord(chord); // your own UI logic
});
await connection.start();
This connects your users to a shared session where the server can push data instantly.
When Should You Use SignalR?
✅ You want live previews (e.g., form updates, dashboards)
✅ You need multi-user awareness (e.g., collaborative music tools or editing)
✅ You’re syncing real-time content (e.g., games, beats, notes, votes)
🔒 Works With ASP.NET Identity, Authorization, and More
You can integrate SignalR seamlessly with:
- Your existing authentication and roles
- API controllers and Razor Pages
- Frontend frameworks like Blazor or plain JavaScript
It’s ready for enterprise use—and already wired up in DevStack with examples for collaborative music tools, file processing queues, and admin dashboards.
Want It Already Working?
SignalR sounds great on paper—but wiring it to your specific app, use case, and authentication model takes time.
Let me help you get there fast:
- Browse the DevStack codebase
- Review my experience in tech and business
- Or book a consult and I’ll plug it in for you
Ready to build a truly live experience?
Let’s make your app feel instant.