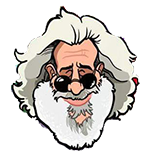
How To Create a Google TXT Sitemap in MVC
All your hard work on your website will be in vain if it is not indexed (and therefore is undiscovered).
This is how to create a google compliant sitemap in text (TXT) format both from records in a database and from a list
Google gives contradictory advice how to do that here https://www.google.com/sitemaps/protocol.html and here https://developers.google.com/search/docs/advanced/sitemaps/build-sitemap
This code creates a file that complies with Google Sitemaps. Add your own Table names, fieldnames etc. and you're good to go!
then submit at https://search.google.com/search-console/sitemaps
Hey, why not do Bing too? https://www.bing.com/webmasters/sitemaps
Alternate link for submit http://google.com/webmaster and http://bing.com/webmaster
public static void SitemapText()
{
// make some declarations
ApplicationDbContext db = new ApplicationDbContext();
var csv = new StringBuilder();
// we have some urls outside of the database too so we'll use Linq to add them from this single dimension string array
List<string> names = new List<string> { "Downloads", "Downloads/SiteMap", "Downloads/?sortOrder=Popular", "Downloads/?sortOrder=Latest", "Downloads/?sortOrder=Name", "Downloads/?sortOrder=Oldest" };
// 1) how to fill from a database table
var downloads = from p in db.Downloads
where p.Flag != true
orderby p.DownloadID descending
select new { p.DownloadID, p.Slug };
foreach (var item in downloads)
{
var newLine = String.Format("https://www.bennysutton.com/downloads/details/{0}-{1}", item.DownloadID, item.Slug); // SEO friendly URL
csv.AppendLine(newLine);
}
// 2) create some elements from the string array
foreach (var name in names)
{
var newLine = String.Format("https://www.bennysutton.com/{0}", name);
csv.AppendLine(newLine);
}
// where to save the file - important to save it at the website root
string savePath = Path.Combine(HttpContext.Current.Server.MapPath("~/"), "sitemap.txt");
// save the doc to text file
string[] foobar = csv.ToString().Split(Environment.NewLine.ToCharArray()); ;
System.IO.File.WriteAllLines(savePath, foobar);
}