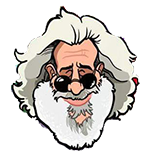
Conditional LINQ queries using C#
A conditional query is one that will accept external parameters 'on the fly'
Never make anything harder than it needs to be. It is easiest to use query syntax, not method syntax to create a conditional query
// your Index action will look something like this...
public ActionResult Index(string searchTerm, int? catID, int? placeID, int page = 1)
{
var query =
from download in db.Downloads
select new // create a new anonymous type that you can fill later
{
download.ID,
download.Name,
// and so on - all the fields you want to consume
};
// logic to build the query according to what is passed in
// i.e. filter on parameters
if (catID.HasValue)
query = query.Where(r => r.CategoryID == catID);
if (placeID.HasValue)
query = query.Where(r => r.PlaceID == placeID);
if (!String.IsNullOrEmpty(searchTerm)) // it's a text search
{
// logic for string search
}
// Now coerce your anonymous type into the type that your razor page consumes
var model = query.Select(r => new PhotoListViewModel
{
Id = r.ID,
Name = r.Name,
// and so on - all the fields you want to consume
})
// logic to return model to the page
}
LINQPad example