c# remove extension from filename
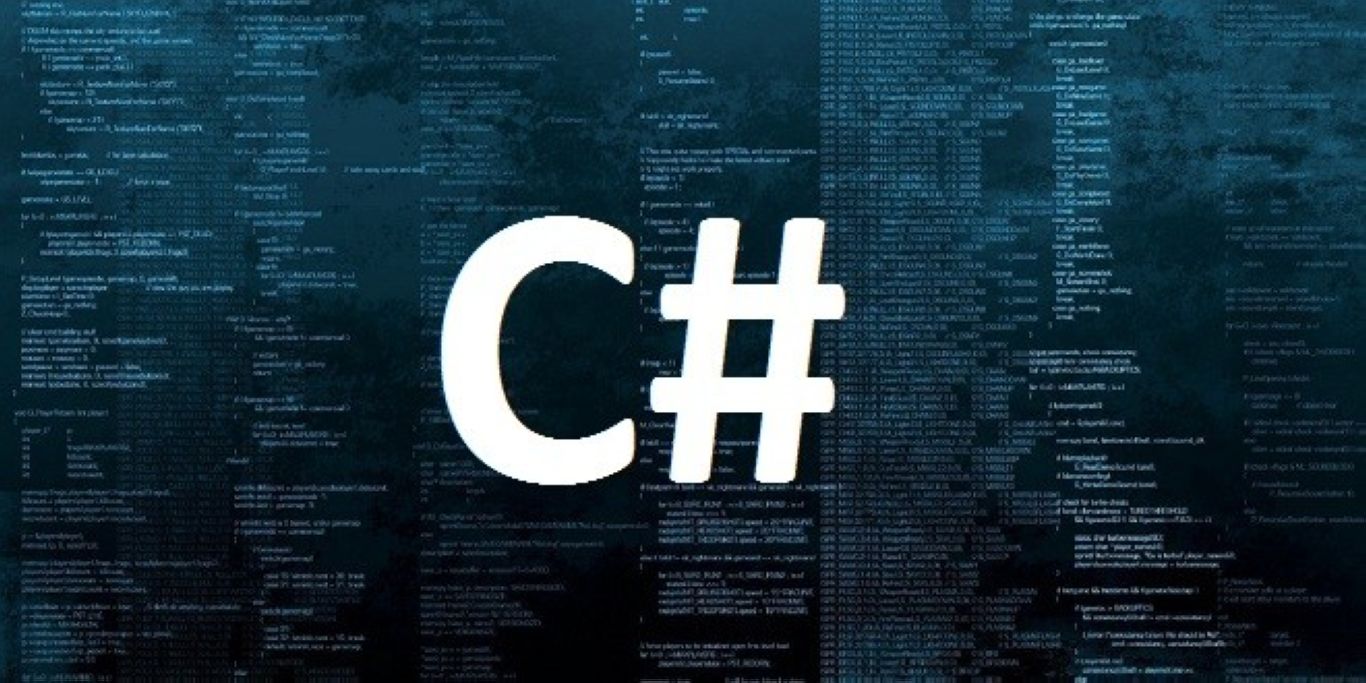
🧠 How to Remove the Extension from a Filename in C#
You can remove the extension from a filename in C# using several methods. Here are two of the most common approaches:
🔧 Using Path.GetFileNameWithoutExtension
You can use the Path.GetFileNameWithoutExtension
method from the System.IO
namespace:
using System.IO;
string fileName = "example.txt";
string fileNameWithoutExtension = Path.GetFileNameWithoutExtension(fileName);
Console.WriteLine(fileNameWithoutExtension); // Output: example
✅ This is the preferred method — clean, built-in, and safe.
✂️ Using Substring
and LastIndexOf
You can also achieve this using raw string manipulation:
string fileName = "example.txt";
int index = fileName.LastIndexOf('.');
string fileNameWithoutExtension = index == -1 ? fileName : fileName.Substring(0, index);
Console.WriteLine(fileNameWithoutExtension); // Output: example
⚠️ This works too but lacks the clarity and robustness of the built-in API.
💡 Final Thought
Both methods will give you the filename without the extension. I recommend using Path.GetFileNameWithoutExtension()
unless you have a very specific reason not to.
Need this logic pre-baked into your utility libraries? It’s already included in my DevStack of .NET Core helpers — so you can focus on building features, not reinventing common code.